"elasticsearch" gem usage
Elastic client initialization ( in config/initializers/elasticsearch.rb ) :
config/initializers/elasticsearch.rb content :
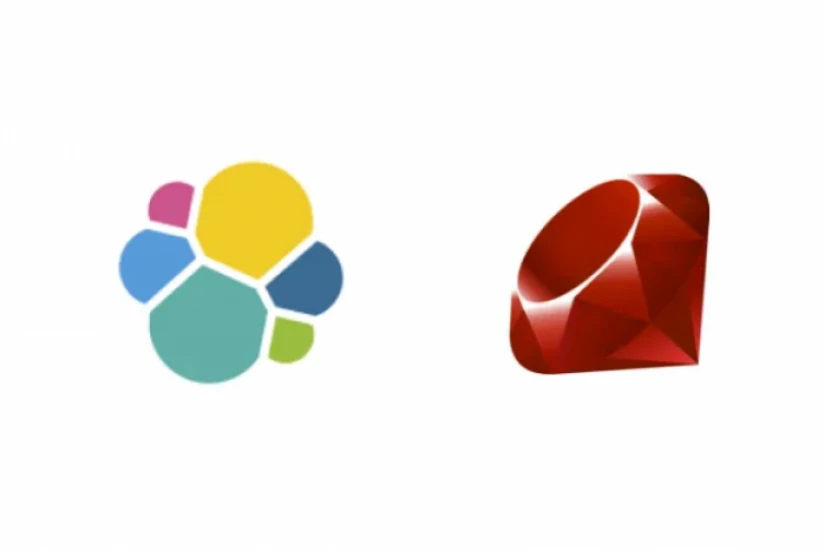
require "elasticsearch"
SearchClient = Elasticsearch::Client.new(
url: ApplicationConfig["ELASTICSEARCH_URL"],
retry_on_failure: 5,
request_timeout: 30,
adapter: :typhoeus,
log: Rails.env.development?,
)
Index creation :
index_settings = { number_of_shards: 1, number_of_replicas: 0 }
settings = { settings: { index: index_settings } }
SearchClient.indices.create(index: "tag_development", body: settings)
Mapping :
MAPPINGS = JSON.parse(File.read("config/elasticsearch/mappings/recipes.json"), symbolize_names: true).freeze recipes.json content :
{ "dynamic": "strict", "properties": { "id": { "type": "keyword" }, "ingredients": { "type": "text", "fields": { "raw": { "type": "keyword" } } } } }SearchClient.indices.put_mapping(index: "tag_development", body: MAPPINGS)
Indexing document :
creating serializer:
module Search
class RecipeSerializer
include FastJsonapi::ObjectSerializer
attributes :id, :ingredients
end
end
adding record to elastic index:
serialized_data = Search::RecipeSerializer.new(record).serializable_hash.dig(:data, :attributes)
SearchClient.index(id: record.id, index: "tag_development", body: serialized_data)
Common query usage :
with logical operators :
SearchClient.search(
index: "tags_development",
body: {
query: {
query_string: {
query: "NOT rausvieji-svogunai",
fields: [ "ingredients" ]
}
}
}
)
return all documents(max 10000):
SearchClient.search(
index: "tag_development",
body: {
query: {
match_all: {}
}
}
)
get by id:
SearchClient.get(id: record.id, index: "tag_development")